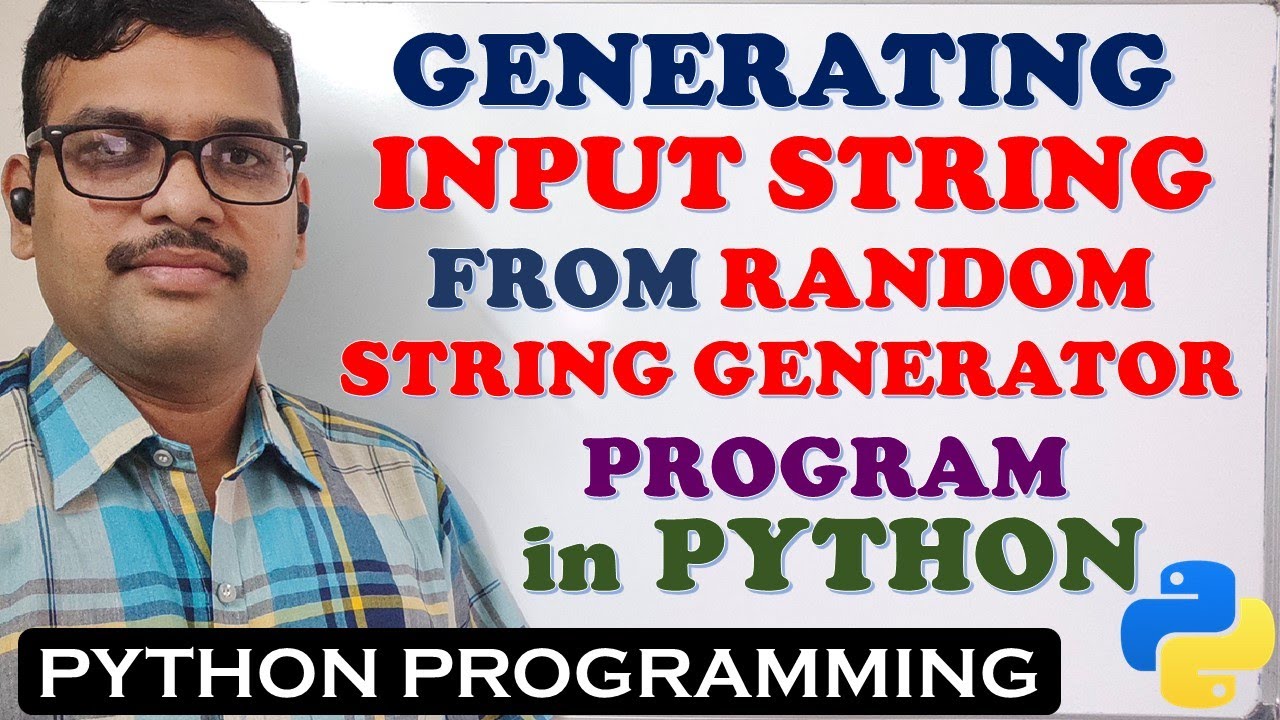
PROGRAM TO GENERATE INPUT STRING FROM RANDOM STRING GENERATOR IN PYTHON PYTHON PROGRAMMING
import string def sec (n): alphabet = string.ascii_letters + string.digits. return ''.join (secrets.choice (alphabet) for i in range (n)) That's it! You've now learned how to create a pseudo-random string, now you just need to choose the module wisely! Python.

Python 3 Random Module Peatix
Method 1: Generate a random string using random.choices () This random.choices () function of a random module can help us achieve this task, and provides a one-liner alternative to a whole loop that might be required for this particular task. Works with Python > v3.6. String.ascii_uppercase - It returns the string with uppercase.
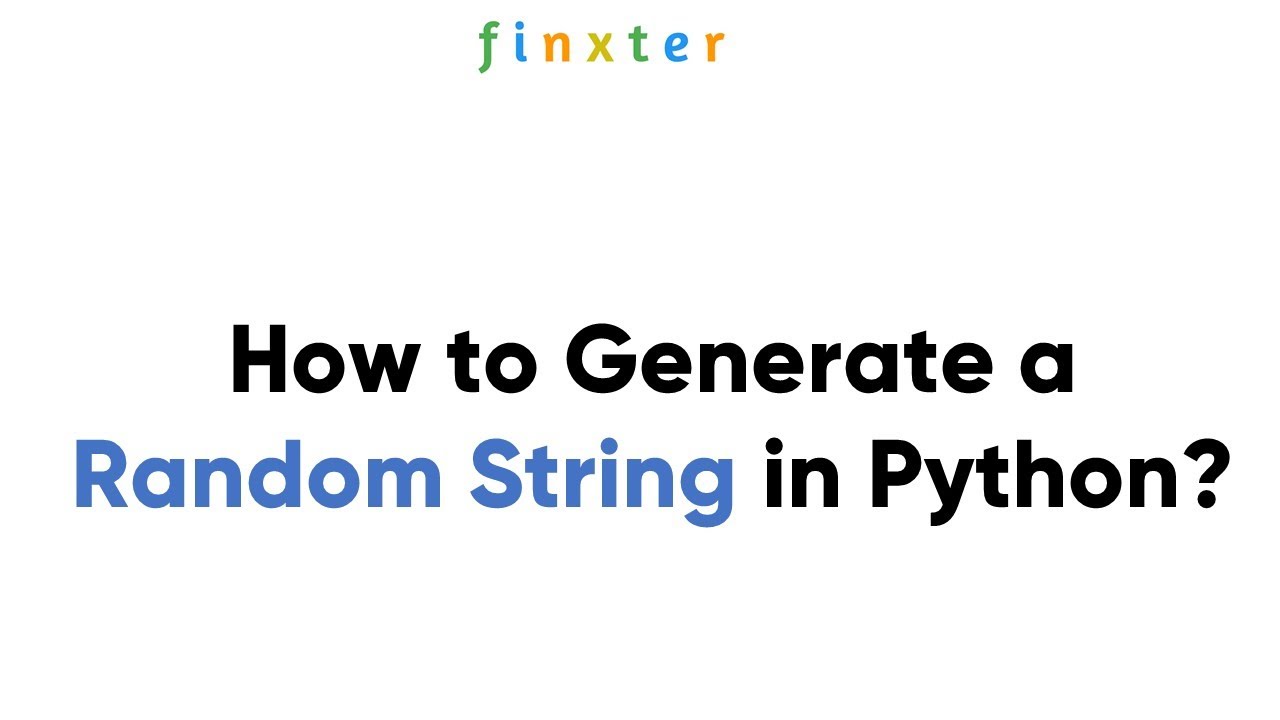
How to Generate a Random String in Python? YouTube
In order to generate random strings in Python, we use the string and random modules. The string module contains Ascii string constants in various text cases, digits, etc. The random module on the other hand is used to generate pseudo-random values.
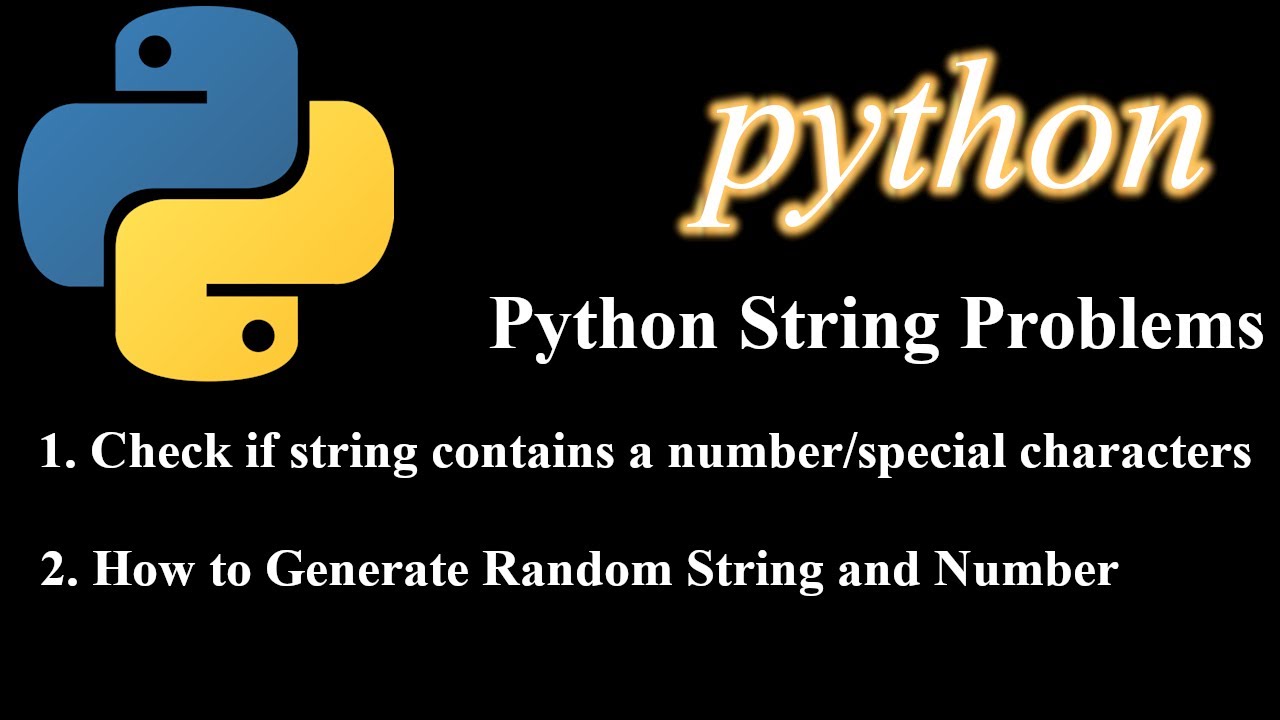
Check if string contain number/special characterGenerate Random StringPython String Problems
import random import string # Set the lenght of the random string length = 20 # Change the value of this variable to your desired length # Define the characters to choose from characters = string.ascii_letters + string.digits # Generate the random string random_string = ''.join(random.choices(characters, k=length)) # Print the random string prin.
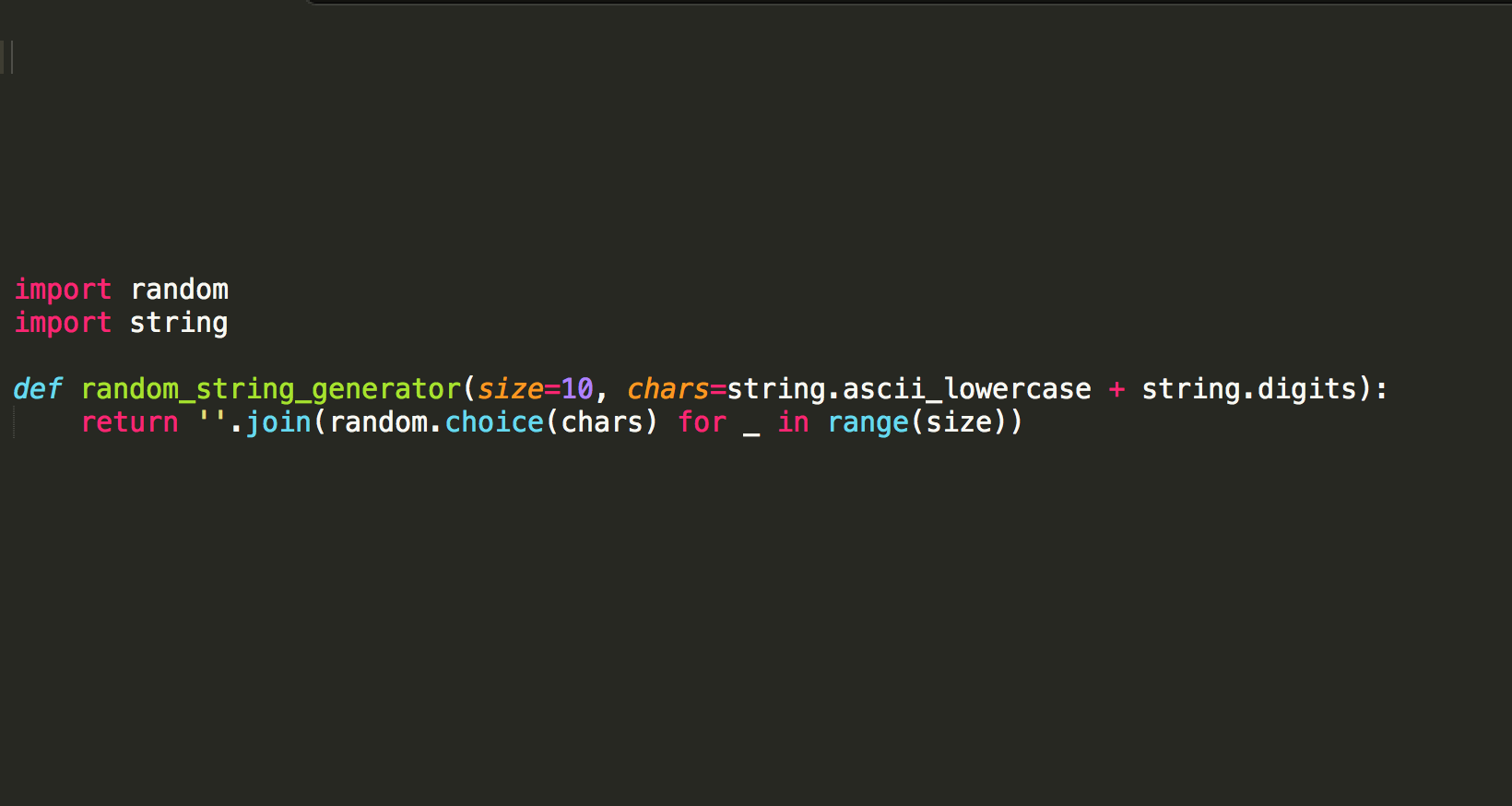
Random String Generator in Python Coding For Entrepreneurs
To generate a random string of specific length, follow these steps. 1. Choose Character Group. Choose the character groups from which you would like to pickup the characters. string class provides following character groups: 2. Call random.choice () Use random.choice () function with all the character groups (appended by + operator) passed as.
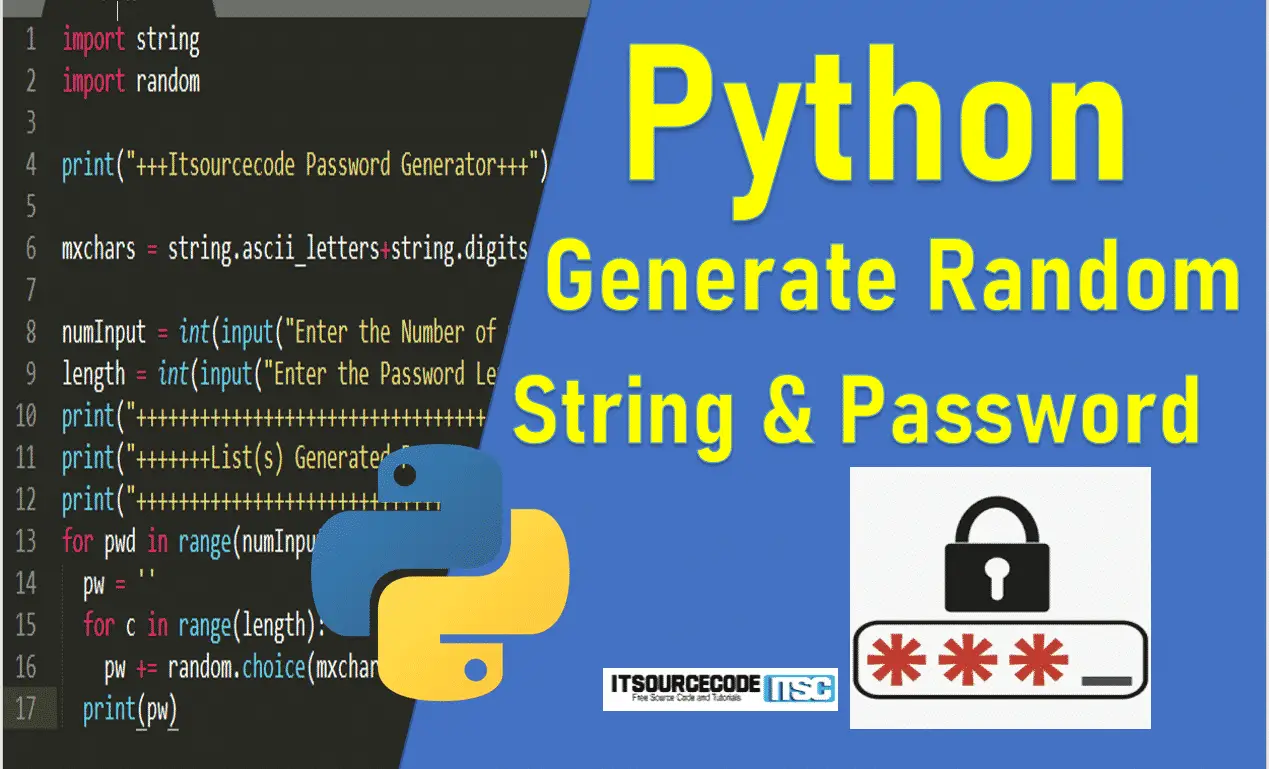
Python Generate Random String and Password with Source Code
13 Answers Sorted by: 273 Generating strings from (for example) lowercase characters: import random, string def randomword (length): letters = string.ascii_lowercase return ''.join (random.choice (letters) for i in range (length)) Results: >>> randomword (10) 'vxnxikmhdc' >>> randomword (10) 'ytqhdohksy' Share Improve this answer Follow

Python Generate Random String With Examples [latest] All Learning
If you need to generate a cryptographically secure random string with special characters, use the secrets module. # Generate a random String with special characters using secrets.choice() This is a three-step process: Use the string module to get a string of special characters, letters and digits.; Use the secrets.choice() method to get N random characters from the string.
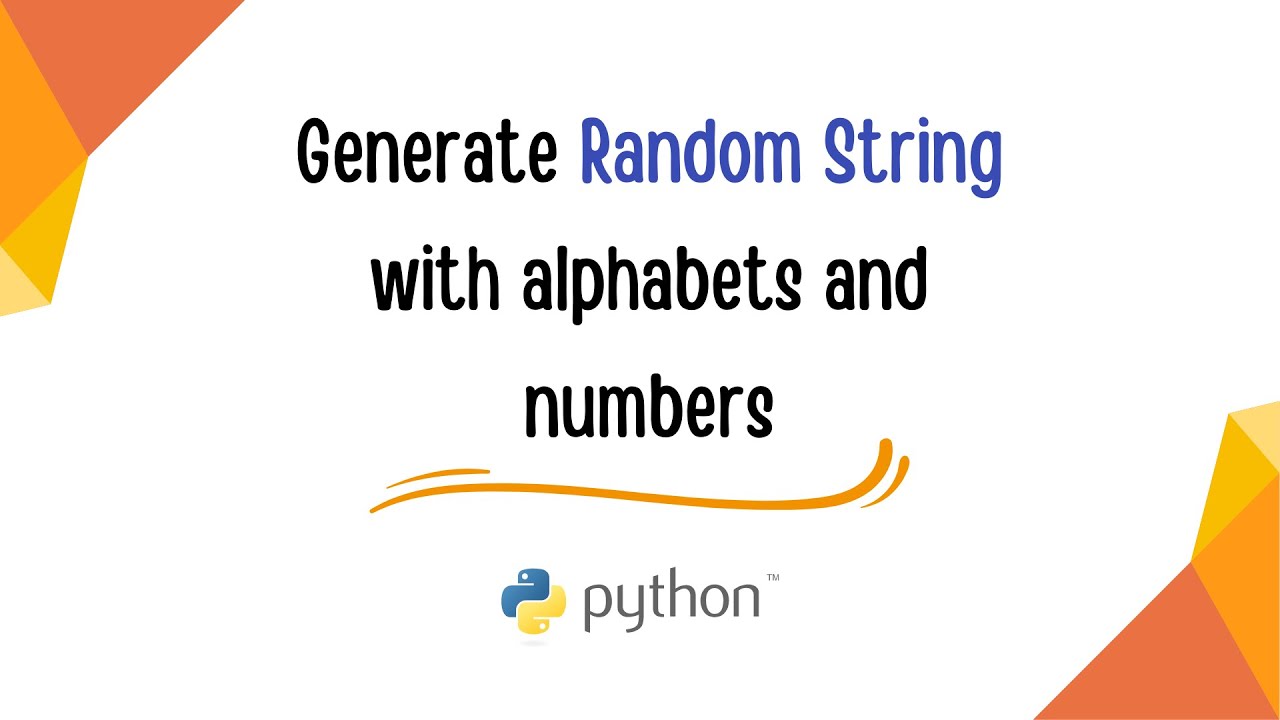
Python Generate Random String with letters and numbers YouTube
Learn how to generate random strings using Python, a useful skill for tasks like password generation or test data creation. LambdaTest Black Friday Sale Is On: Save 30%off on selected annual plans. Use Coupon code: BFCM30 Platform Online Browser Testing Manual live-interactive cross browser testing Selenium Testing
How to Generate Random Data in Python Python Code
First method we will be using to create a random string of combination of upper case letters and digits is the choice () method of random module, which comes bundled with Python. We will use string.ascii_uppercase () and string.digits () functions of string method to genarate random alphabets and digits respectively.
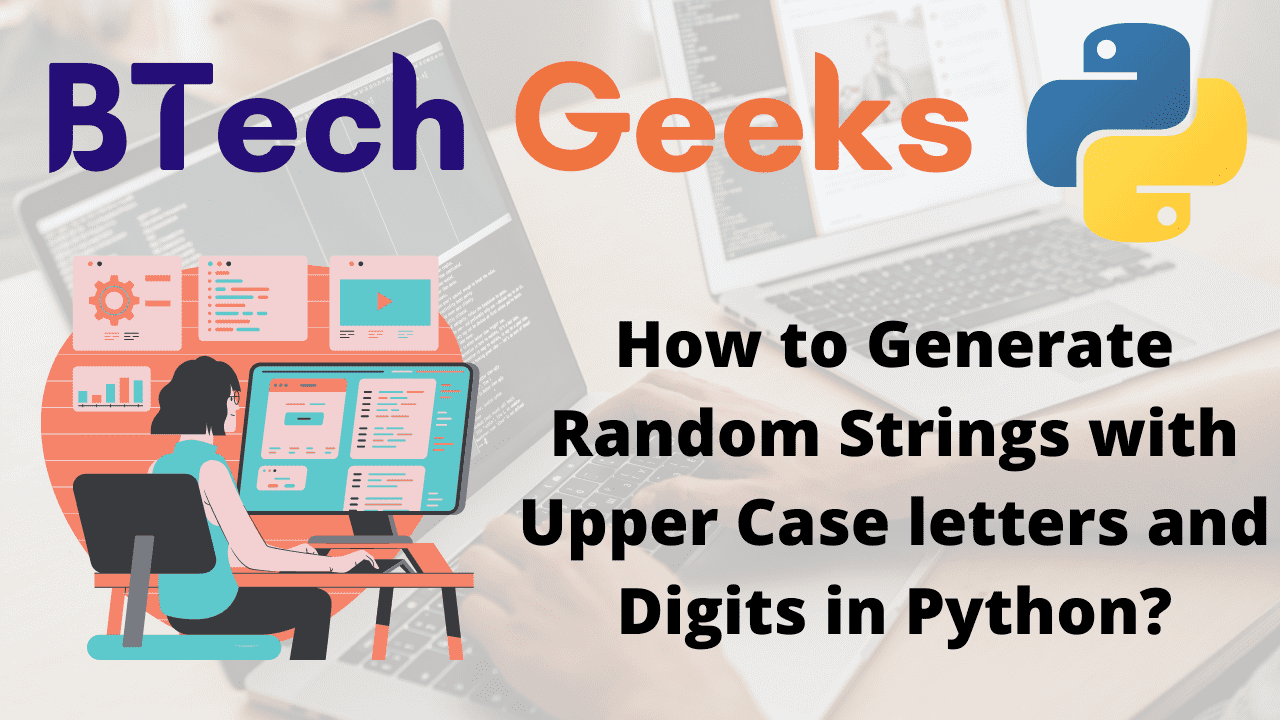
How to Generate Random Strings with Upper Case letters and Digits in Python? BTech Geeks
In order to generate random strings in Python, we use the string and random modules. The string module contains Ascii string constants in various text cases, digits, etc. The random module on the other hand is used to generate pseudo-random values.
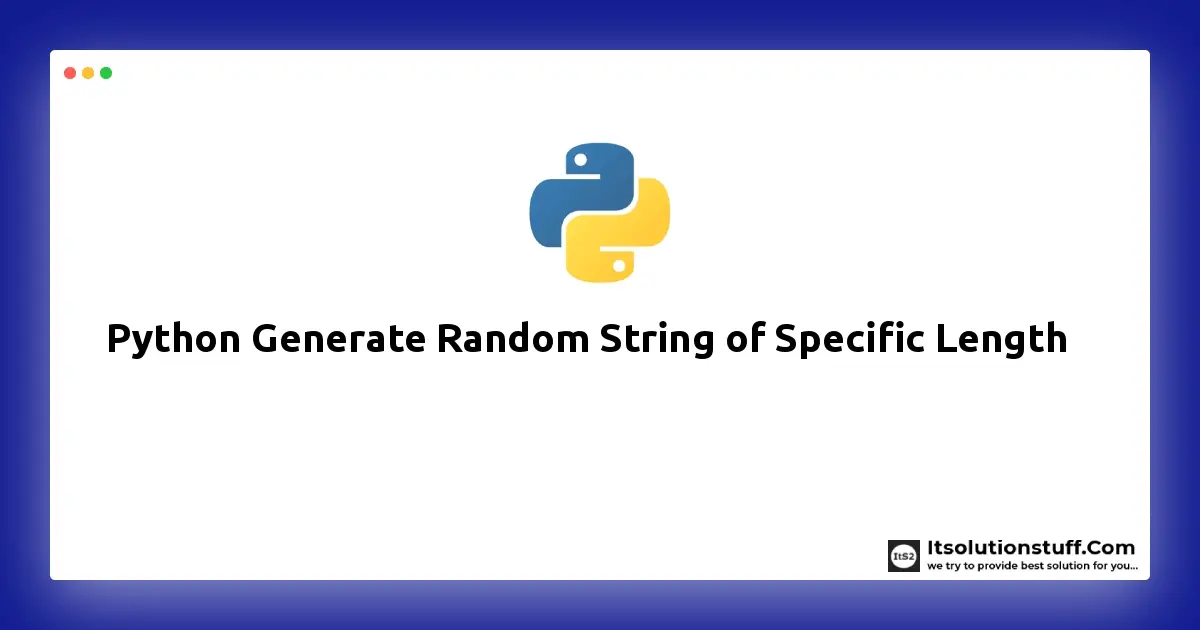
Python Generate Random String of Specific Length Example
There are 3 solutions (and probably 100 more): The"classic" for method appending everyone of the N=4 letters to the same string and the choice method of the random module that picks one element among its arguments, Using the join method to add in one time the elements generated by the choices method of the random module. The choices method is very versatile and can be used with several.

How to Generate a Random String in Python? Finxter
How to Create a Random String in Python Example to generate a random string of any length Random String of Lower Case and Upper Case Letters Random string of specific letters Random String without Repeating Characters Create Random Password with Special characters, letters, and digits
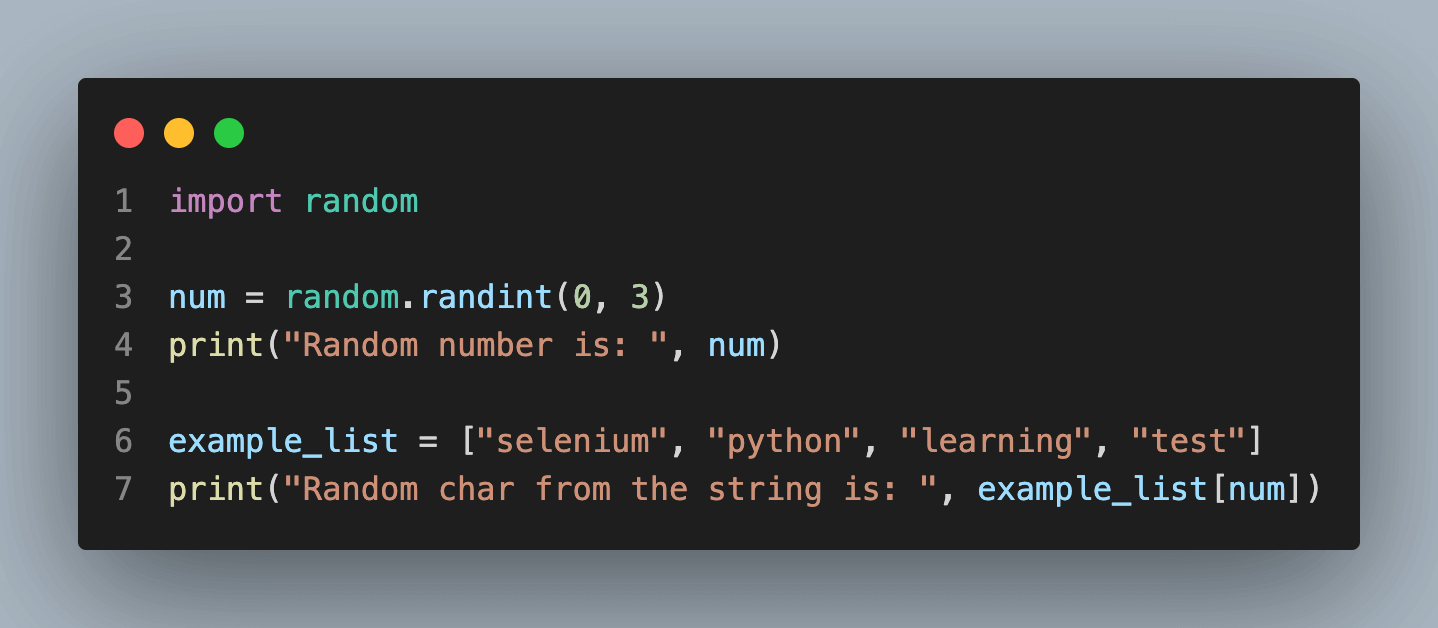
How To Use Python For Random String Generation
Explanation: The 'string.ascii_lowercase' string constant contains all the lowercase letters of the alphabet. The 'random.choice()' function selects a random character from the specified sequence, in this case 'string.ascii_lowercase'. The resulting character is assigned to the variable 'random_char' and printed to the console. You can generate random characters from other.
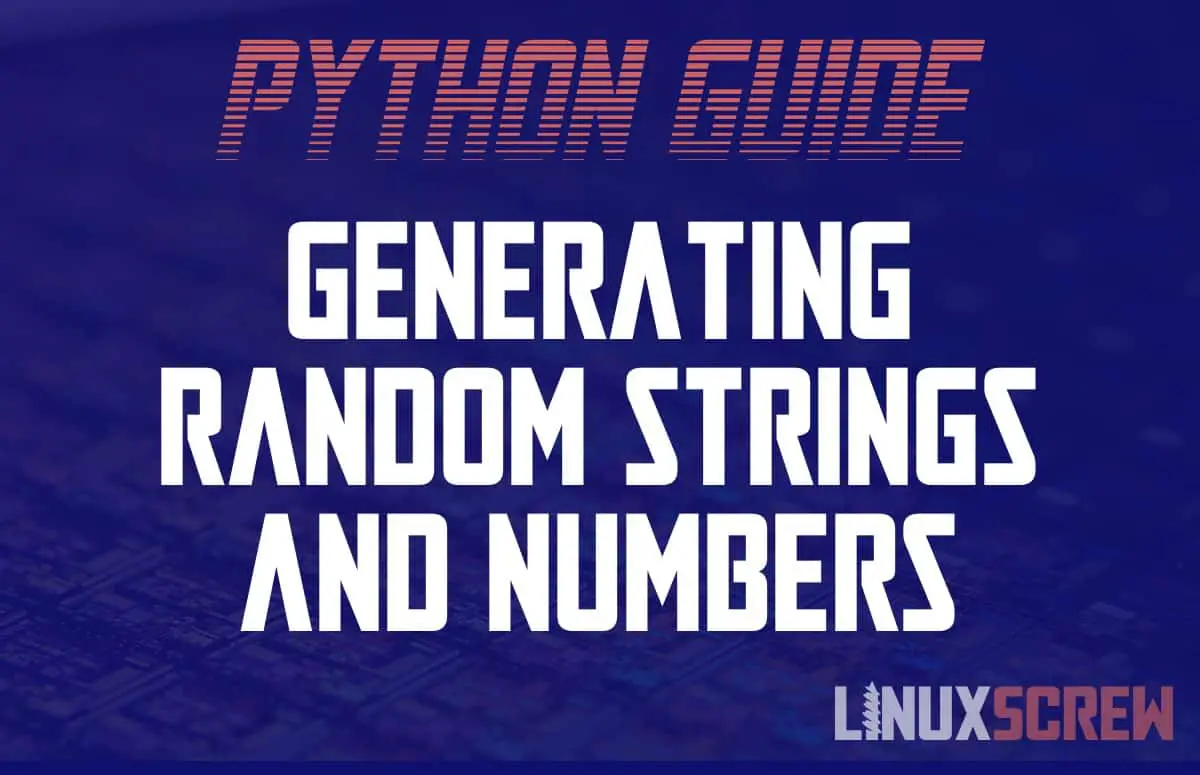
How to Generate Random Numbers and Strings in Python
To generate a random string in Python, we need to provide the sequence of characters from which we want our code to generate the random string to the random.choice () method. The input sequence can consist of capital alphabets, small alphabets, digits and punctuation marks, etc.
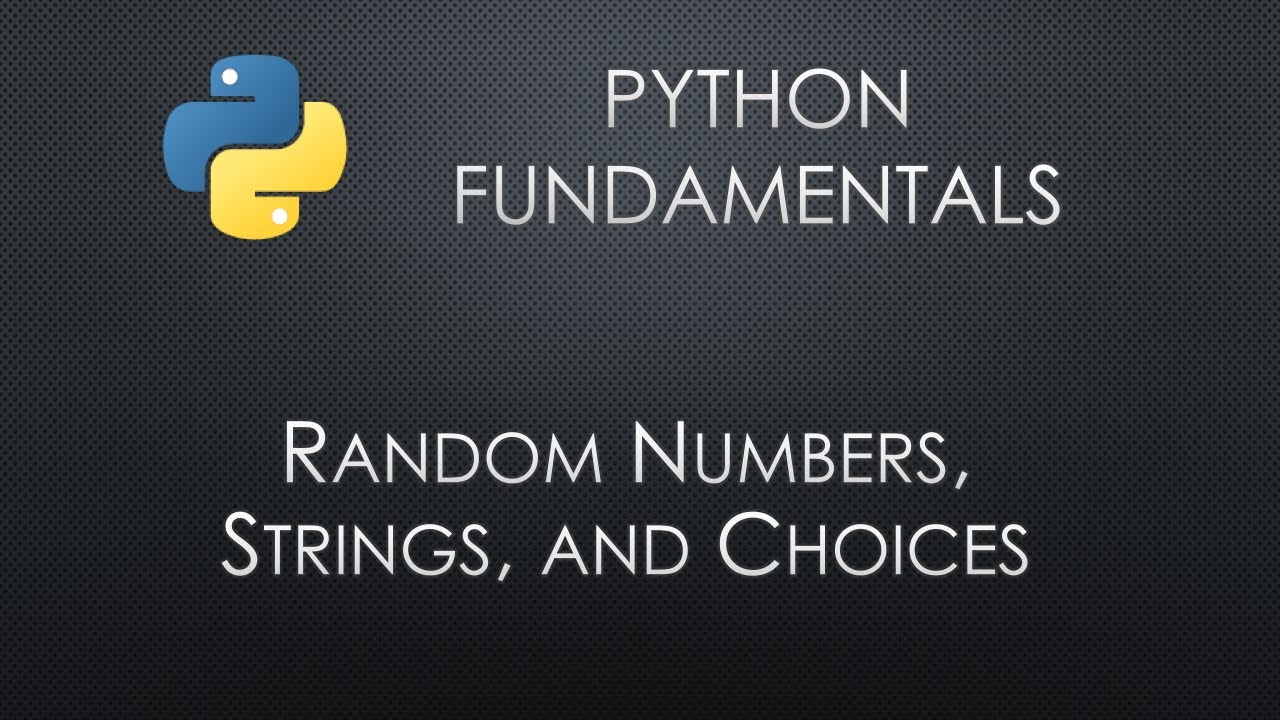
Python Random Numbers, Strings, and Choices YouTube
To generate a random string in Python, you can use the random module and the string module. Here is an example of how to generate a random string of length 10, containing only lowercase letters: import random import string def generate_random_string(length): return ''.join(random.choices(string.ascii_lowercase, k=length)) random_string.

Python Snippets How to Generate Random String Python Program for Generation Random String or
How random is random? This is a weird question to ask, but it is one of paramount importance in cases where information security is concerned. Whenever you're generating random data, strings, or numbers in Python, it's a good idea to have at least a rough idea of how that data was generated.